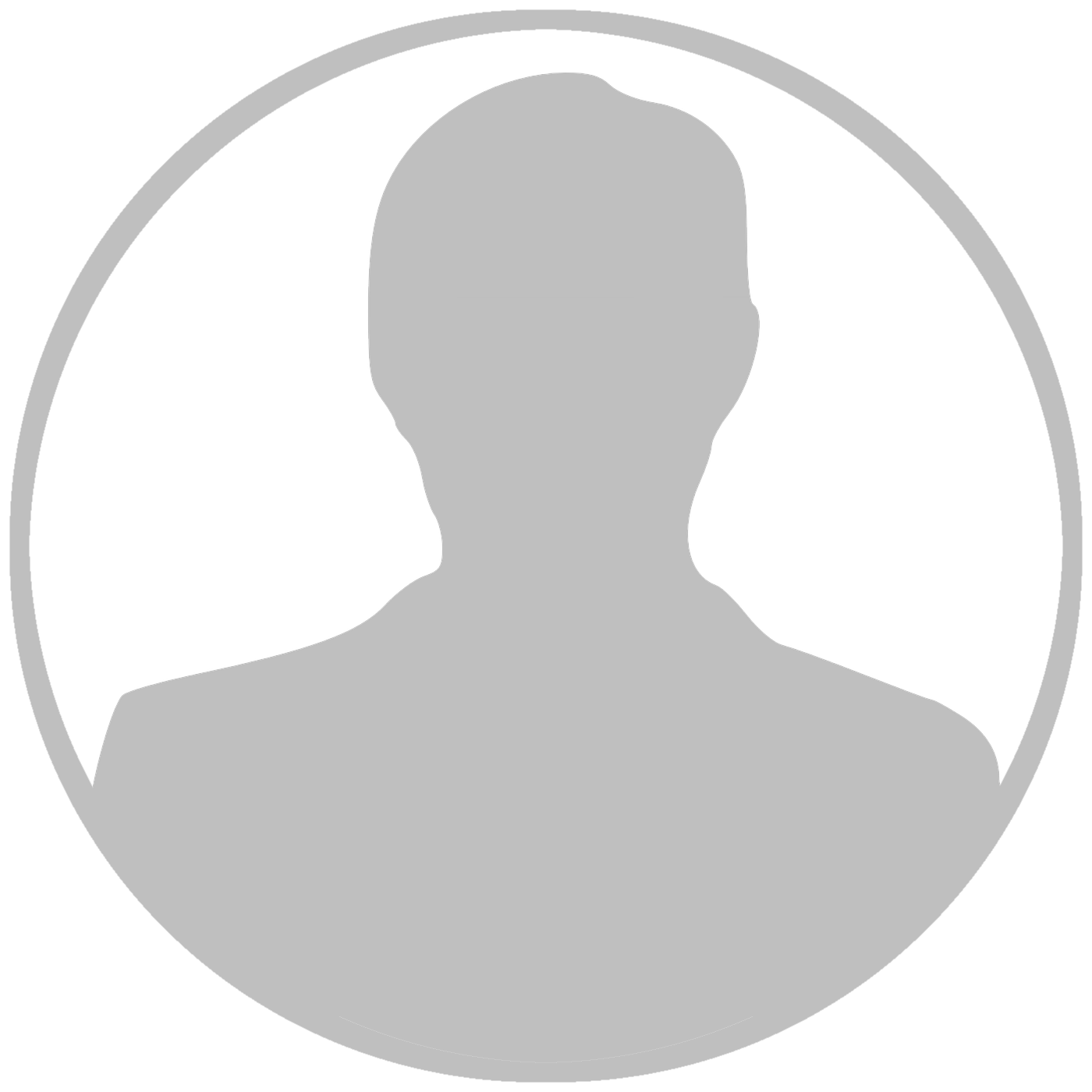
- Support for exponential backoff on reconnect to be gentle on the server. - Clean up client by moving metrics and retry strategy to the connection manager. - Update echo_client to use client manager - Fix echo client XMPP message matching Fixes #21 Improvements for #8
25 lines
636 B
Go
25 lines
636 B
Go
package xmpp_test
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"gosrc.io/xmpp"
|
|
)
|
|
|
|
func TestDurationForAttempt_NoJitter(t *testing.T) {
|
|
b := xmpp.Backoff{Base: 25, NoJitter: true}
|
|
bInMS := time.Duration(b.Base) * time.Millisecond
|
|
if b.DurationForAttempt(0) != bInMS {
|
|
t.Errorf("incorrect default duration for attempt #0 (%d) = %d", b.DurationForAttempt(0)/time.Millisecond, bInMS/time.Millisecond)
|
|
}
|
|
var prevDuration, d time.Duration
|
|
for i := 0; i < 10; i++ {
|
|
d = b.DurationForAttempt(i)
|
|
if !(d >= prevDuration) {
|
|
t.Errorf("duration should be increasing between attempts. #%d (%d) > %d", i, d, prevDuration)
|
|
}
|
|
prevDuration = d
|
|
}
|
|
}
|